Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
制作树¶
这是一个关于如何从头开始制作树和其他类型植被的小教程.
The aim is to not focus on the modeling techniques (there are plenty of tutorials about that), but how to make them look good in Godot.
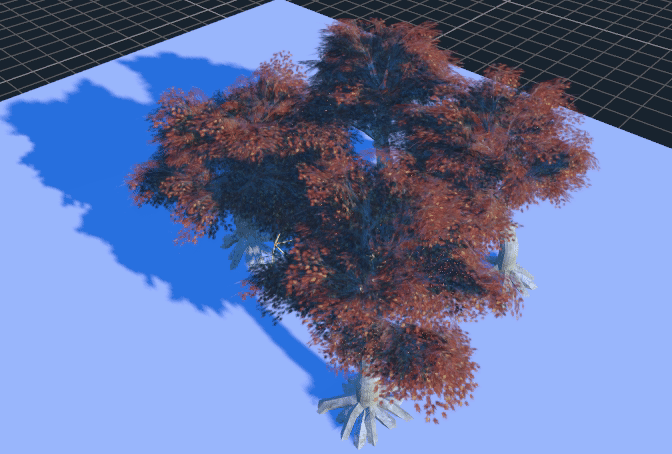
从一棵树开始¶
我从 SketchFab 上获取了这棵树:
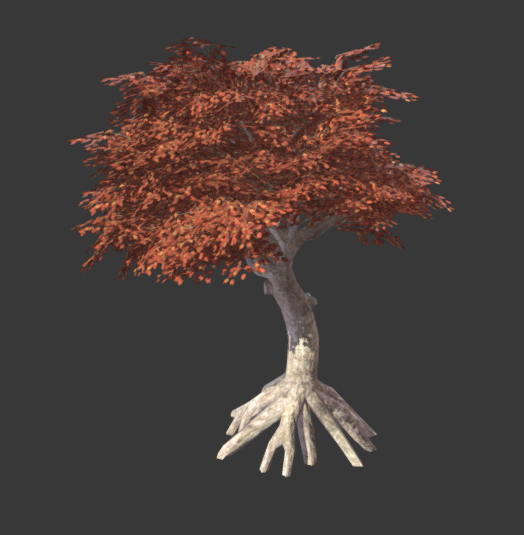
https://sketchfab.com/models/ea5e6ed7f9d6445ba69589d503e8cebf
然后用 Blender 打开。
用顶点颜色绘制¶
The first thing you may want to do is to use the vertex colors to paint how much the tree will sway when there is wind. Just use the vertex color painting tool of your favorite 3D modeling program and paint something like this:
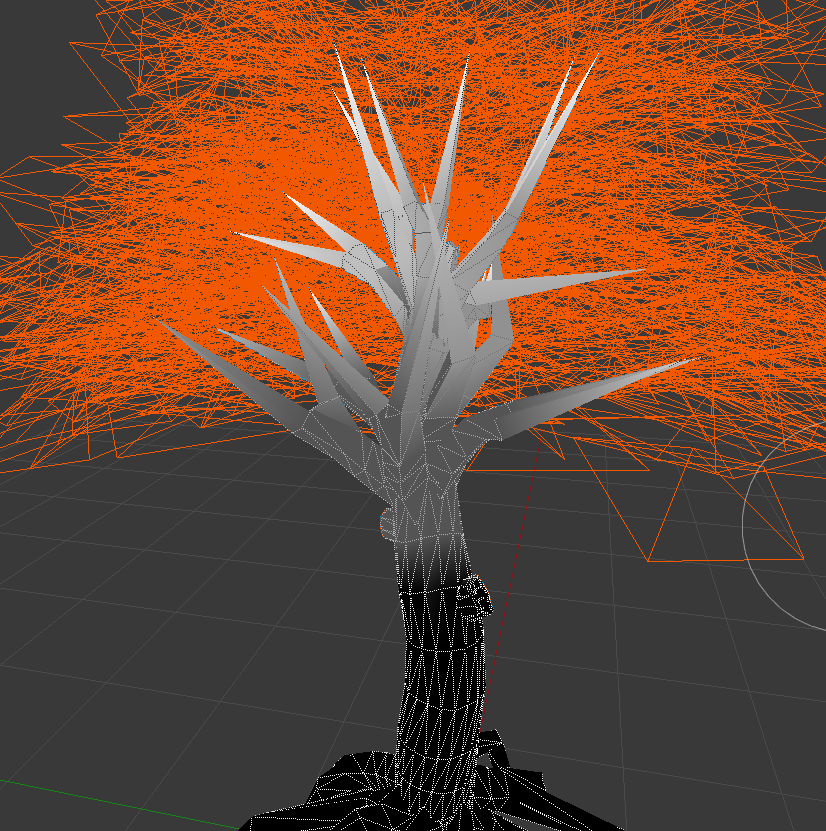
这有点夸张, 但这个想法是, 颜色表明了多少摇摆影响树的每个部分. 这个比例尺更能说明问题:
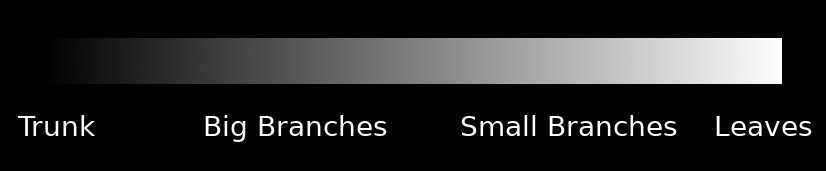
为叶子编写自定义着色器¶
This is an example of a shader for leaves:
shader_type spatial;
render_mode depth_prepass_alpha, cull_disabled, world_vertex_coords;
这是一个空间着色器. 没有前/后剔除(所以可以从两边看到叶子), 并且使用了alpha预通道, 所以使用透明度(和叶子投射阴影)导致的深度伪影比较少. 最后, 对于摇摆的效果, 推荐使用世界坐标, 以使树可以被复制, 移动等, 并且仍然可以和其他树一起使用.
uniform sampler2D texture_albedo : source_color;
uniform vec4 transmission : source_color;
在这里, 纹理和透射颜色被读取, 透射颜色被用来给叶子添加一些背光, 模拟地下散射.
uniform float sway_speed = 1.0;
uniform float sway_strength = 0.05;
uniform float sway_phase_len = 8.0;
void vertex() {
float strength = COLOR.r * sway_strength;
VERTEX.x += sin(VERTEX.x * sway_phase_len * 1.123 + TIME * sway_speed) * strength;
VERTEX.y += sin(VERTEX.y * sway_phase_len + TIME * sway_speed * 1.12412) * strength;
VERTEX.z += sin(VERTEX.z * sway_phase_len * 0.9123 + TIME * sway_speed * 1.3123) * strength;
}
这是创建叶子摆动的代码. 它是基本的(只是使用正弦波乘以时间和轴的位置, 但工作得很好). 注意, 强度乘以颜色. 每个轴使用不同的小的接近1.0的乘法系数, 所以轴不同步出现.
最后, 剩下的就是片段着色器了:
void fragment() {
vec4 albedo_tex = texture(texture_albedo, UV);
ALBEDO = albedo_tex.rgb;
ALPHA = albedo_tex.a;
METALLIC = 0.0;
ROUGHNESS = 1.0;
TRANSMISSION = transmission.rgb;
}
差不多就是这样.
主干着色器是类似的, 除了它不写到alpha通道(因此不需要alpha前置)和不需要传输工作. 这两个着色器都可以通过添加法线映射, AO和其他映射来改进.
改进着色器¶
还有更多的资源可以做到这一点, 你可以阅读. 现在你已经了解了基础知识, 建议阅读GPU Gems3中关于Crysis如何做到这一点的章节(主要关注摇摆代码, 因为许多其他技术都已经过时了):
https://developer.nvidia.com/gpugems/GPUGems3/gpugems3_ch16.html