Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
Creating the enemy¶
Nadszedł czas, aby stworzyć przeciwników, których nasz gracz będzie musiał unikać. Ich zachowanie nie będzie zbyt skomplikowane: przeciwnicy będą pojawiać się losowo na krawędziach ekranu i poruszać w losowym kierunku po linii prostej, a następnie usuwać się, gdy "wyjdą" poza granicę ekranu po przeciwnej stronie.
Stworzymy scenę ``Mob``(Przeciwnik), której będziemy mogli tworzyć instancje, czyli powielać ją dowolną razy, tworząc niezależnych przeciwników w grze.
Konfiguracja węzła¶
Click Scene -> New Scene from the top menu and add the following nodes:
RigidBody2D (o nazwie
Mob
)
Nie zapomnij ustawić dzieci tak, aby nie mogły być wybierane, tak jak zrobiliśmy to w przypadku sceny z graczem.
Select the Mob
node and set its Gravity Scale
property in the RigidBody2D
section of the inspector to 0
.
This will prevent the mob from falling downwards.
In addition, under the CollisionObject2D
section just beneath the RigidBody2D section,
expand the Collision group and
uncheck the 1
inside the Mask
property.
This will ensure the mobs do not collide with each other.
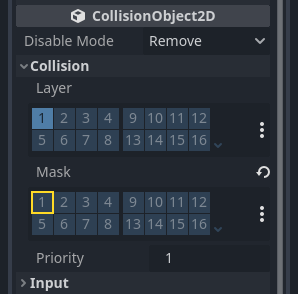
Set up the AnimatedSprite2D like you did for the
player. This time, we have 3 animations: fly
, swim
, and walk
. There
are two images for each animation in the art folder.
The Animation Speed
property has to be set for each individual animation. Adjust it to 3
for all 3 animations.
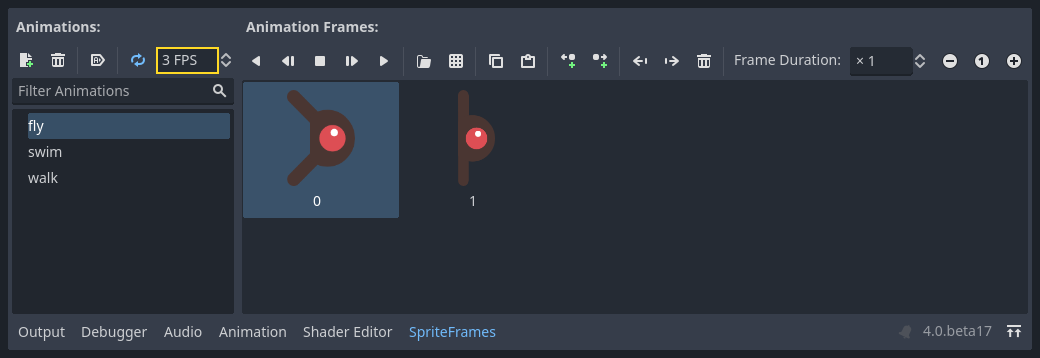
You can use the "Play Animation" buttons on the right of the Animation Speed
input field to preview your animations.
Będziemy wybrać jedną z tych animacji losowo, stąd nasi przeciwnicy będą w jakiś sposób różnorodni.
Like the player images, these mob images need to be scaled down. Set the
AnimatedSprite2D
's Scale
property to (0.75, 0.75)
.
As in the Player
scene, add a CapsuleShape2D
for the collision. To align
the shape with the image, you'll need to set the Rotation
property
to 90
(under "Transform" in the Inspector).
Zapisz scenę.
Skrypt wroga¶
Add a script to the Mob
like this:
extends RigidBody2D
using Godot;
public partial class Mob : RigidBody2D
{
// Don't forget to rebuild the project.
}
Now let's look at the rest of the script. In _ready()
we play the animation
and randomly choose one of the three animation types:
func _ready():
var mob_types = $AnimatedSprite2D.sprite_frames.get_animation_names()
$AnimatedSprite2D.play(mob_types[randi() % mob_types.size()])
public override void _Ready()
{
var animatedSprite2D = GetNode<AnimatedSprite2D>("AnimatedSprite2D");
string[] mobTypes = animatedSprite2D.SpriteFrames.GetAnimationNames();
animatedSprite2D.Play(mobTypes[GD.Randi() % mobTypes.Length]);
}
First, we get the list of animation names from the AnimatedSprite2D's sprite_frames
property. This returns an Array containing all three animation names: ["walk",
"swim", "fly"]
.
Następnie uzyskujemy losową wartość pomiędzy 0
a 2
, aby na jej podstawie móc uzyskać nazwę znajdującą się na wylosowanym miejscu na liście (tablica zaczyna liczenie miejsc od 0
- to znaczy, że na naszej liście nazwa walk
kryje się pod wartością 0
, nazwa swim
pod 1
, zaś nazwa fly
pod 2
). Funkcja randi() % n
wybiera losową liczbę pomiędzy zerem, a n-1
.
The last piece is to make the mobs delete themselves when they leave the screen.
Connect the screen_exited()
signal of the VisibleOnScreenNotifier2D
node
to the Mob
and add this code:
func _on_visible_on_screen_notifier_2d_screen_exited():
queue_free()
private void OnVisibleOnScreenNotifier2DScreenExited()
{
QueueFree();
}
To kończy tworzenie sceny Mob.
With the player and enemies ready, in the next part, we'll bring them together in a new scene. We'll make enemies spawn randomly around the game board and move forward, turning our project into a playable game.