Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
Interpolation(補間)¶
補間は、グラフィックプログラミングの非常に基本的な操作です。グラフィックス開発者としての視野を広げるために、これに慣れることは良いことです。
基本的な考え方は、AからBに移行することです。値 t
は、中間の状態を表します。
For example, if t
is 0, then the state is A. If t
is 1, then the state is B. Anything in-between is an interpolation.
Between two real (floating-point) numbers, an interpolation can be described as:
interpolation = A * (1 - t) + B * t
多くの場合、次のように簡略化されます:
interpolation = A + (B - A) * t
The name of this type of interpolation, which transforms a value into another at constant speed is "linear". So, when you hear about Linear Interpolation, you know they are referring to this formula.
他のタイプの補間がありますが、ここでは説明しません。後で Bezier のページを読むことをお勧めします。
ベクトル補間¶
Vector types (Vector2 and Vector3) can also be interpolated, they come with handy functions to do it Vector2.lerp() and Vector3.lerp().
For cubic interpolation, there are also Vector2.cubic_interpolate() and Vector3.cubic_interpolate(), which do a Bezier style interpolation.
Here is example pseudo-code for going from point A to B using interpolation:
var t = 0.0
func _physics_process(delta):
t += delta * 0.4
$Sprite2D.position = $A.position.lerp($B.position, t)
private float _t = 0.0f;
public override void _PhysicsProcess(double delta)
{
_t += (float)delta * 0.4f;
Marker2D a = GetNode<Marker2D>("A");
Marker2D b = GetNode<Marker2D>("B");
Sprite2D sprite = GetNode<Sprite2D>("Sprite2D");
sprite.Position = a.Position.Lerp(b.Position, _t);
}
次のモーションを生成します:
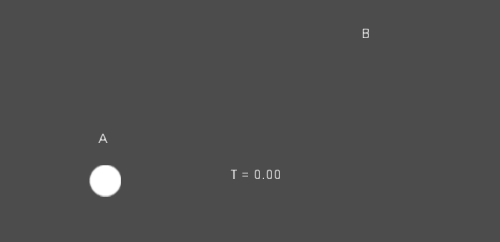
幾何学変換(変形)の補間¶
It is also possible to interpolate whole transforms (make sure they have either uniform scale or, at least, the same non-uniform scale). For this, the function Transform3D.interpolate_with() can be used.
以下は、猿をPosition1からPosition2に変換する例です:
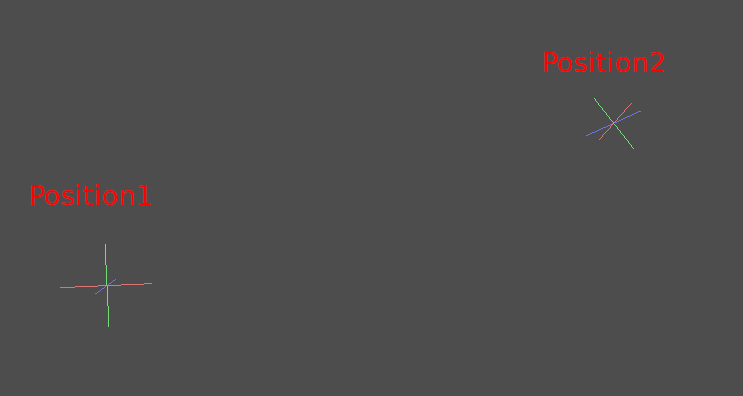
次の擬似コードを使用します:
var t = 0.0
func _physics_process(delta):
t += delta
$Monkey.transform = $Position1.transform.interpolate_with($Position2.transform, t)
private float _t = 0.0f;
public override void _PhysicsProcess(double delta)
{
_t += (float)delta;
Marker3D p1 = GetNode<Marker3D>("Position1");
Marker3D p2 = GetNode<Marker3D>("Position2");
CSGMesh3D monkey = GetNode<CSGMesh3D>("Monkey");
monkey.Transform = p1.Transform.InterpolateWith(p2.Transform, _t);
}
また、次のモーションを生成します:
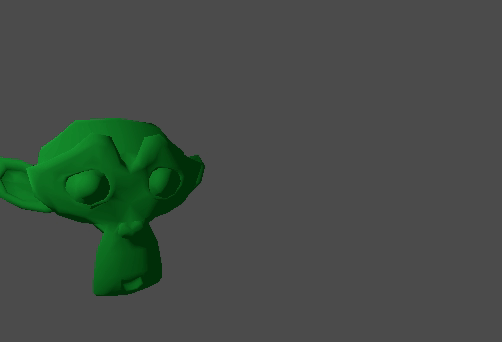
スムージングモーション¶
補間は、動きや回転などを滑らかにするために使用できます。これは、滑らかな動きを使用したマウスに追従する円の例です:
const FOLLOW_SPEED = 4.0
func _physics_process(delta):
var mouse_pos = get_local_mouse_position()
$Sprite2D.position = $Sprite2D.position.lerp(mouse_pos, delta * FOLLOW_SPEED)
private const float FollowSpeed = 4.0f;
public override void _PhysicsProcess(double delta)
{
Vector2 mousePos = GetLocalMousePosition();
Sprite2D sprite = GetNode<Sprite2D>("Sprite2D");
sprite.Position = sprite.Position.Lerp(mousePos, (float)delta * FollowSpeed);
}
次の様に見えます:
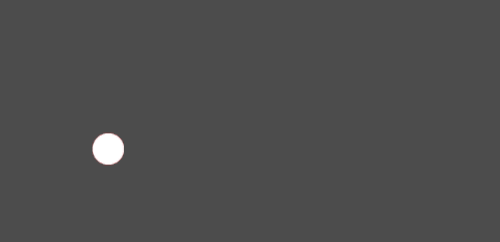
これは、カメラの動きを滑らかにしたり、仲間を追いかけたり(一定範囲内にとどまるようにしたり)、その他多くの一般的なゲームパターンに役立ちます。