Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
Noções básicas de C#¶
Introdução¶
This page provides a brief introduction to C#, both what it is and how to use it in Godot. Afterwards, you may want to look at how to use specific features, read about the differences between the C# and the GDScript API, and (re)visit the Scripting section of the step-by-step tutorial.
C# is a high-level programming language developed by Microsoft. In Godot, it is implemented with .NET 6.0.
Atenção
Projects written in C# using Godot 4 currently cannot be exported to the web platform. To use C# on the web platform, consider Godot 3 instead. Android and iOS platform support is available as of Godot 4.2, but is experimental and some limitations apply.
Nota
Esse não é um tutorial completo e abrangente sobre a linguagem C#. Se você ainda não estiver familiarizado com sua sintaxe ou recursos, consulte o guia C# da Microsoft ou procure uma introdução adequada em outro lugar.
Pré-requisitos¶
Godot bundles the parts of .NET needed to run already compiled games. However, Godot does not bundle the tools required to build and compile games, such as MSBuild and the C# compiler. These are included in the .NET SDK, and need to be installed separately.
In summary, you must have installed the .NET SDK and the .NET-enabled version of Godot.
Download and install the latest stable version of the SDK from the .NET download page.
Importante
Certifique-se de instalar a versão de 64 bits do(s) SDK(s) se estiver usando a versão de 64 bits do Godot.
If you are building Godot from source, make sure to follow the steps to enable .NET support in your build as outlined in the Compiling with .NET page.
Configurando um editor externo¶
C# support in Godot's built-in script editor is minimal. Consider using an external IDE or editor, such as Visual Studio Code or MonoDevelop. These provide autocompletion, debugging, and other useful features for C#. To select an external editor in Godot, click on Editor → Editor Settings and scroll down to Dotnet. Under Dotnet, click on Editor, and select your external editor of choice. Godot currently supports the following external editors:
Visual Studio 2022
Visual Studio Code
MonoDevelop
Visual Studio para Mac
JetBrains Rider
Veja as seções a seguir sobre como configurar um editor externo:
JetBrains Rider¶
Após ler a seção "Pré-requisitos", você pode baixar e instalar o JetBrains Rider.
No menu Editor → Configurações do Editor do Godot:
Set Dotnet -> Editor -> External Editor to JetBrains Rider.
No Rider:
Defina a versão do MSBuild para .NET Core.
Instale o plugin de suporte do Godot.
Visual Studio Code¶
Após ler a seção "Pré-requisitos", você pode baixar e instalar o Visual Studio Code (também conhecido como VS Code).
No menu Editor → Configurações do Editor do Godot:
Set Dotnet -> Editor -> External Editor to Visual Studio Code.
No Visual Studio Code:
Instale a extensão C#.
Nota
Se você está usando Linux, você precisa instalar o Mono SDK <https://www.mono-project.com/download/stable/#download-lin> para que o plugin de ferramentas C# funcione.
To configure a project for debugging, you need a tasks.json
and launch.json
file in
the .vscode
folder with the necessary configuration. An example configuration can be
found here .
In the launch.json
file, make sure the program
parameter in the relevant configuration points to your Godot executable, either by
changing it to the path of the executable or by defining a GODOT4
environment variable that points to the
executable. Now, when you start the debugger in Visual Studio Code, your Godot project will run.
Nota
There is also a C# Tools for Godot Visual Studio Code extension, that is meant to make this setup easier and to provide further useful tools. But it is not yet updated to work with Godot 4.
Visual Studio (somente Windows)¶
Baixe e instale a última versão do Visual Studio. O Visual Studio incluirá os SDKs necessários se você tiver as cargas de trabalho corretas selecionadas, para que você não precise instalar manualmente as coisas listadas na seção "Pré-requisitos".
While installing Visual Studio, select this workload:
.NET desktop development
No menu Editor → Configurações do Editor do Godot:
Set Dotnet -> Editor -> External Editor to Visual Studio.
Nota
If you see an error like "Unable to find package Godot.NET.Sdk", your NuGet configuration may be incorrect and need to be fixed.
A simple way to fix the NuGet configuration file is to regenerate it.
In a file explorer window, go to %AppData%\NuGet
. Rename or delete
the NuGet.Config
file. When you build your Godot project again,
the file will be automatically created with default values.
Criando um script C#¶
Depois de configurar com sucesso o C# para Godot, você deverá ver a seguinte opção ao selecionar Attach Script no menu de contexto de um nó em sua cena:
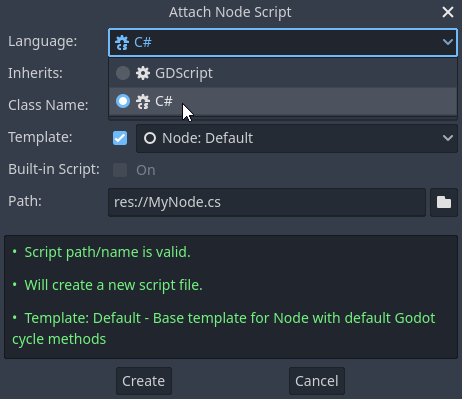
Note that while some specifics change, most concepts work the same when using C# for scripting. If you're new to Godot, you may want to follow the tutorials on Linguagens de script at this point. While some documentation pages still lack C# examples, most notions can be transferred from GDScript.
Configuração de projeto e fluxo de trabalho¶
When you create the first C# script, Godot initializes the C# project files
for your Godot project. This includes generating a C# solution (.sln
)
and a project file (.csproj
), as well as some utility files and folders
(.godot/mono
).
All of these but .godot/mono
are important and should be committed to your
version control system. Everything under .godot
can be safely added to the
ignore list of your VCS.
When troubleshooting, it can sometimes help to delete the .godot/mono
folder
and let it regenerate.
Exemplo¶
Aqui está um script C# em branco com alguns comentários para demonstrar como funciona.
using Godot;
public partial class YourCustomClass : Node
{
// Member variables here, example:
private int _a = 2;
private string _b = "textvar";
public override void _Ready()
{
// Called every time the node is added to the scene.
// Initialization here.
GD.Print("Hello from C# to Godot :)");
}
public override void _Process(double delta)
{
// Called every frame. Delta is time since the last frame.
// Update game logic here.
}
}
As you can see, functions normally in global scope in GDScript like Godot's
print
function are available in the GD
static class which is part of
the Godot
namespace. For a full list of methods in the GD
class, see the
class reference pages for
@GDScript and @GlobalScope.
Nota
Keep in mind that the class you wish to attach to your node should have the same
name as the .cs
file. Otherwise, you will get the following error:
"Cannot find class XXX for script res://XXX.cs"
Diferenças gerais entre o C# e o GDScript¶
A API do C# usa o PascalCase
em vez do snake_case
no GDScript/C++. Sempre que possível, fields e getters/setters foram convertidos em propriedades. Em geral, a API C# Godot se esforça para ser tão idiomática quanto for razoavelmente possível.
Para mais informações, veja a página Diferenças da API C# para GDScript.
Aviso
You need to (re)build the project assemblies whenever you want to see new exported variables or signals in the editor. This build can be manually triggered by clicking the Build button in the top right corner of the editor.

Você também precisará reconstruir os assemblies do projeto para aplicar alterações nos scripts de "ferramenta".
Pegadas gerais e problemas conhecidos¶
As C# support is quite new in Godot, there are some growing pains and things that need to be ironed out. Below is a list of the most important issues you should be aware of when diving into C# in Godot, but if in doubt, also take a look over the official issue tracker for .NET issues.
Escrever plugins para o editor é possível, mas atualmente é bastante complicado.
Atualmente o estado não é salvo e restaurado durante o "hot-reloading", com exceção das variáveis exportadas.
Os scripts C# anexados devem se referir a uma classe que tenha um nome de classe que corresponda ao nome do arquivo.
There are some methods such as
Get()
/Set()
,Call()
/CallDeferred()
and signal connection methodConnect()
that rely on Godot'ssnake_case
API naming conventions. So when using e.g.CallDeferred("AddChild")
,AddChild
will not work because the API is expecting the originalsnake_case
versionadd_child
. However, you can use any custom properties or methods without this limitation. Prefer using the exposedStringName
in thePropertyName
,MethodName
andSignalName
to avoid extraStringName
allocations and worrying about snake_case naming.
As of Godot 4.0, exporting .NET projects is supported for desktop platforms (Linux, Windows and macOS). Other platforms will gain support in future 4.x releases.
Common pitfalls¶
You might encounter the following error when trying to modify some values in Godot
objects, e.g. when trying to change the X coordinate of a Node2D
:
public partial class MyNode2D : Node2D
{
public override _Ready()
{
Position.X = 100.0f;
// CS1612: Cannot modify the return value of 'Node2D.Position' because
// it is not a variable.
}
}
This is perfectly normal. Structs (in this example, a Vector2
) in C# are
copied on assignment, meaning that when you retrieve such an object from a
property or an indexer, you get a copy of it, not the object itself. Modifying
said copy without reassigning it afterwards won't achieve anything.
The workaround is simple: retrieve the entire struct, modify the value you want to modify, and reassign the property.
var newPosition = Position;
newPosition.X = 100.0f;
Position = newPosition;
Since C# 10, it is also possible to use with expressions on structs, allowing you to do the same thing in a single line.
Position = Position with { X = 100.0f };
You can read more about this error on the C# language reference.
Desempenho do C# no Godot¶
According to some preliminary benchmarks, the performance of C# in Godot — while generally in the same order of magnitude — is roughly ~4× that of GDScript in some naive cases. C++ is still a little faster; the specifics are going to vary according to your use case. GDScript is likely fast enough for most general scripting workloads.
Most properties of Godot C# objects that are based on GodotObject
(e.g. any Node
like Control
or Node3D
like Camera3D
) require native (interop) calls as they talk to
Godot's C++ core.
Consider assigning values of such properties into a local variable if you need to modify or read them multiple times at
a single code location:
using Godot;
public partial class YourCustomClass : Node3D
{
private void ExpensiveReposition()
{
for (var i = 0; i < 10; i++)
{
// Position is read and set 10 times which incurs native interop.
// Furthermore the object is repositioned 10 times in 3D space which
// takes additional time.
Position += new Vector3(i, i);
}
}
private void Reposition()
{
// A variable is used to avoid native interop for Position on every loop.
var newPosition = Position;
for (var i = 0; i < 10; i++)
{
newPosition += new Vector3(i, i);
}
// Setting Position only once avoids native interop and repositioning in 3D space.
Position = newPosition;
}
}
Passing raw arrays (such as byte[]
) or string
to Godot's C# API requires marshalling which is
comparatively pricey.
The implicit conversion from string
to NodePath
or StringName
incur both the native interop and marshalling
costs as the string
has to be marshalled and passed to the respective native constructor.
Usando pacotes NuGet no Godot¶
Pacotes NuGet podem ser instalados e usados com o Godot, como qualquer projeto. Muitas IDEs podem adicionar pacotes diretamente. Eles também podem ser adicionados manualmente adicionando a referência do pacote no arquivo .csproj
localizado na pasta principal do projeto:
<ItemGroup>
<PackageReference Include="Newtonsoft.Json" Version="11.0.2" />
</ItemGroup>
...
</Project>
A partir da versão 3.2.3, o Godot automaticamente baixa e instala os últimos pacotes NuGet na próxima vez que ele compila o projeto.
Perfilando seu código C#¶
The following tools may be used for performance and memory profiling of your managed code:
JetBrains Rider with dotTrace/dotMemory plugin.
Standalone JetBrains dotTrace/dotMemory.
Visual Studio.
Profiling managed and unmanaged code at once is possible with both JetBrains tools and Visual Studio, but limited to Windows.