Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
Monitor de alerta¶
A peça final que nosso jogo precisa é uma Interface de Usuário (UI) para exibir coisas como pontuação, uma mensagem de "game over" e um botão de reiniciar.
Create a new scene, click the "Other Node" button and add a CanvasLayer node named
HUD
. "HUD" stands for "heads-up display", an informational display that
appears as an overlay on top of the game view.
O nó CanvasLayer nos permite desenhar nossos elementos de interface em uma camada acima do resto do jogo, de forma que as informações que ela mostrar não fiquem cobertas por quaisquer elementos do jogo, como o jogador ou os inimigos.
O HUD exibirá as seguintes informações:
Pontuação, alterado para
ScoreTimer
.Uma mensagem, como "Game Over" ou "Prepare-se!"
Um botão "Iniciar" para começar o jogo.
O nó básico para elementos de interface é Control. Para criar nossa interface, usaremos dois tipos de nós Control: Label e Button.
Crie os seguintes itens como filhos do nó HUD
:
Label nomeado
ScoreLabel
.Label nomeado
MessageLabel
.Button nomeado
StartButton
.Timer nomeado
MessageTimer
.
Clique no ScoreLabel
e digite um número no campo Text``no Inspetor. A fonte padrão para os nós ``Control
é pequena e não escala bem. Há um arquivo de fonte incluído nos assets do jogo chamado "Xolonium-Regular.ttf". Para usar esta fonte, faça o seguinte para cada um dos três nós Control
:
Under "Theme Overrides > Fonts", choose "Load" and select the "Xolonium-Regular.ttf" file.
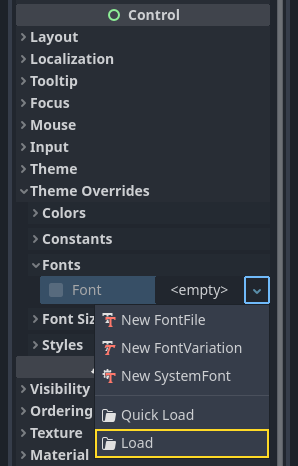
The font size is still too small, increase it to 64
under "Theme Overrides > Font Sizes".
Once you've done this with the ScoreLabel
, repeat the changes for the Message
and StartButton
nodes.
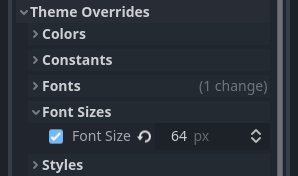
Nota
Anchors: Control
nodes have a position and size,
but they also have anchors. Anchors define the origin -
the reference point for the edges of the node.
Arrange the nodes as shown below. You can drag the nodes to place them manually, or for more precise placement, use "Anchor Presets".
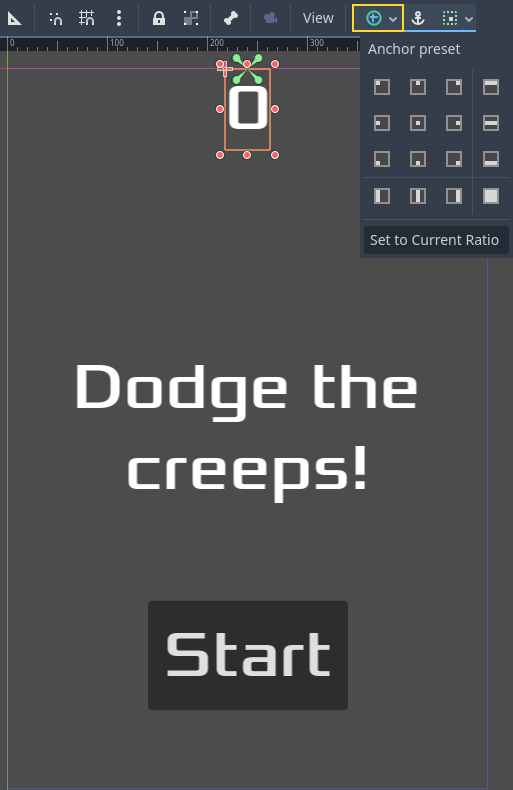
ScoreLabel¶
Adicione o texto
0
.Defina o "Alinhamento Horizontal" e "Alinhamento Vertical" para
Center
.Choose the "Anchor Preset"
Center Top
.
Mensagem¶
Add the text
Dodge the Creeps!
.Defina o "Alinhamento Horizontal" e "Alinhamento Vertical" para
Center
.Defina o "Modo Autowrap" para
Word
, caso contrário o rótulo permanecerá em uma linha.Under "Control - Layout/Transform" set "Size X" to
480
to use the entire width of the screen.Choose the "Anchor Preset"
Center
.
Conectando HUD a Principal¶
Agora que terminamos de criar a cena HUD
, salve-a e volte para a Principal
. Crie uma instância da cena HUD
como fez com a cena Jogador
, e coloque-a no final da árvore. A árvore completa deveria se parecer assim, então confira se não falta alguma coisa:
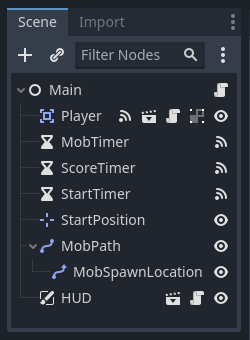
Agora precisamos conectar a funcionalidade de HUD
ao roteiro de Principal
. Isso exige algumas adições à cena Principal
:
In the Node tab, connect the HUD's start_game
signal to the new_game()
function of the Main node by clicking the "Pick" button in the "Connect a Signal"
window and selecting the new_game()
method or type "new_game" below "Receiver Method"
in the window. Verify that the green connection icon now appears next to
func new_game()
in the script.
Em new_game()
, atualize o mostrador de pontuação e mostre a mensagem "Prepare-se":
$HUD.update_score(score)
$HUD.show_message("Get Ready")
var hud = GetNode<HUD>("HUD");
hud.UpdateScore(_score);
hud.ShowMessage("Get Ready!");
Em game_over()
, precisamos chamar a correspondente função de HUD
:
$HUD.show_game_over()
GetNode<HUD>("HUD").ShowGameOver();
Finally, add this to _on_score_timer_timeout()
to keep the display in sync
with the changing score:
$HUD.update_score(score)
GetNode<HUD>("HUD").UpdateScore(_score);
Aviso
Remember to remove the call to new_game()
from
_ready()
if you haven't already, otherwise
your game will start automatically.
Now you're ready to play! Click the "Play the Project" button. You will be asked
to select a main scene, so choose main.tscn
.
Removendo antigas criaturas¶
Se você jogar até o "Game Over" e iniciar um novo jogo, as criaturas do jogo anterior ainda poderão estar na tela. Seria melhor se todas elas desaparecessem no iníco de cada partida. Nós só precisamos de um jeito de falar para todos os inimigos se auto-destruirem. Nós podemos fazer isso com a funcionalidade "group"(grupo).
Na cena do Inimigo
, selecione o nó raiz e clique na aba "Nó" próxima ao Inspetor(No mesmo lugar onde vocÊ encontra os sinais do nó). Próximo a "Sinais", clique "Grupos", você pode digitar o nome do novo grupo e clicar em "Adicionar".
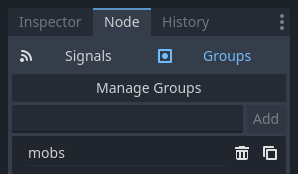
Agora todos os inimigos estarão no grupo "inimigos". Podemos então adicionar a seguinte linha à função game_over()
em Main
:
get_tree().call_group("mobs", "queue_free")
// Note that for calling Godot-provided methods with strings,
// we have to use the original Godot snake_case name.
GetTree().CallGroup("mobs", Node.MethodName.QueueFree);
A função ``call_group()``chama a função passada como parâmetro em cada nó do grupo - neste caso nós estamos falando para cada inimigo se auto-destruir.
O jogo está quase pronto nesse ponto. Na próxima e última parte, daremos um melhor acabamento adicionando um plano de fundo, música em "looping", e alguns atalhos de teclado.