Work in progress
The content of this page was not yet updated for Godot
4.2
and may be outdated. If you know how to improve this page or you can confirm
that it's up to date, feel free to open a pull request.
スクリプト言語¶
このレッスンではGodotで使用できるスクリプト言語の概要をお伝えします。それぞれの長所と短所も学習できます。次のパートではGDScriptを使って最初のスクリプトを書いていきます。
スクリプトはノードに接続され、ノードの振る舞いを拡張するもの です。これは接続されるノードの関数とプロパティすべてを継承することを意味します。
例えば、Camera2Dノードが船を追いかけるゲームで考えてみましょう。Camera2Dノードは、デフォルトでは親ノードについて動きます。ここで、プレイヤーがダメージを受けると、カメラが揺れるようにしたいとします。この機能はGodotに組み込まれていないので、スクリプトをアタッチしてカメラが揺れるようにコーディングする必要があります。
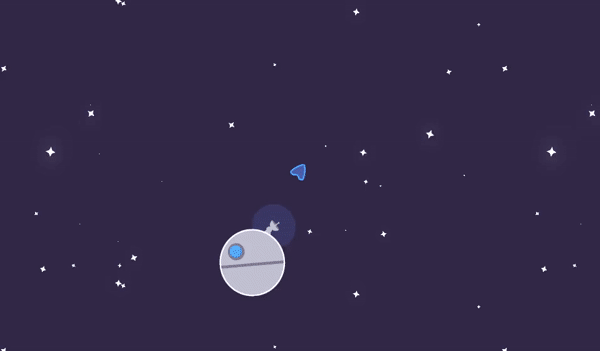
利用可能なスクリプト言語¶
Godot offers four gameplay programming languages: GDScript, C#, and, via its GDExtension technology, C and C++. There are more community-supported languages, but these are the official ones.
You can use multiple languages in a single project. For instance, in a team, you could code gameplay logic in GDScript as it's fast to write, and use C# or C++ to implement complex algorithms and maximize their performance. Or you can write everything in GDScript or C#. It's your call.
このように、さまざまなゲームプロジェクトや開発者のニーズに応えるために、柔軟な対応を行っています。
どの言語を使うべきか?¶
もしあなたが初心者なら、GDScriptから始めることをお勧めします。私たちはこの言語を、Godot とゲーム開発者のニーズのために特別に作りました。軽量でわかりやすい構文を持ち、Godot との最も緊密な統合を提供します。
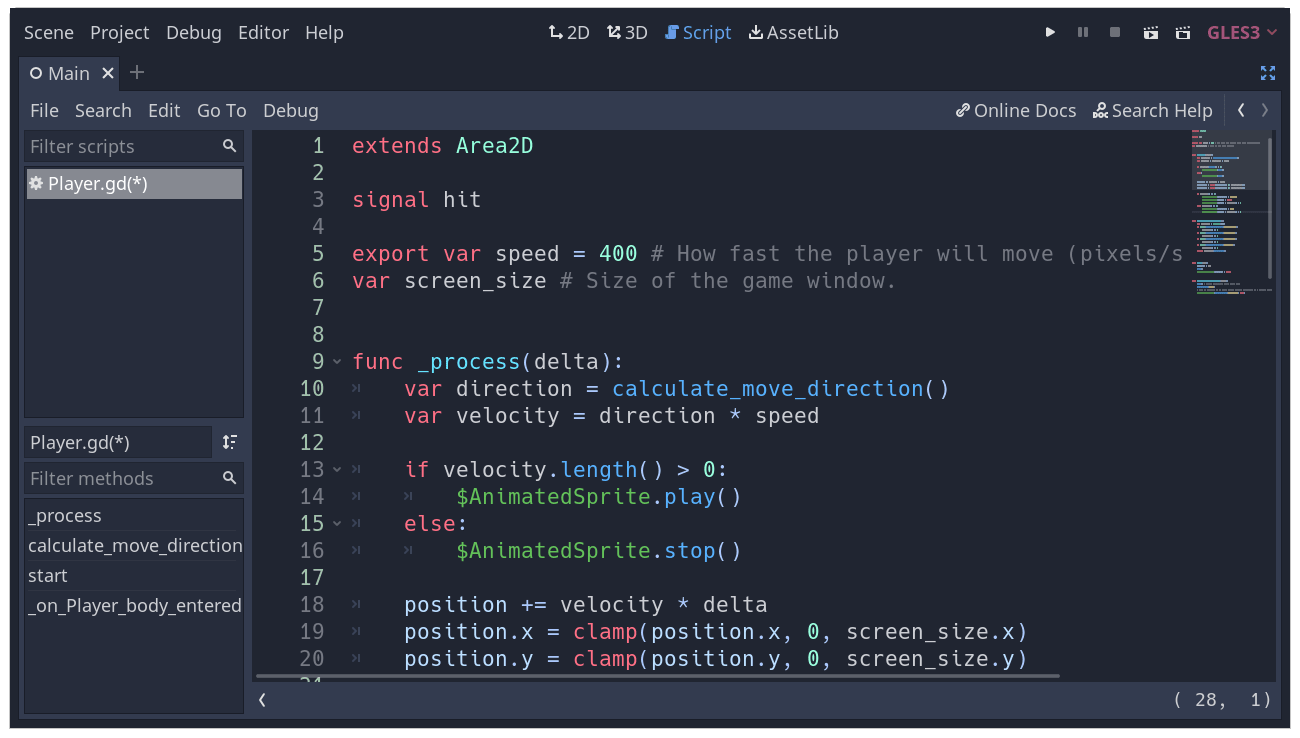
C# の場合は、VSCode や Visual Studio などの外部のコードエディターが必要になります。C# は十分にサポートされていますが、GDScript と比較すると学習に役立つ情報はあまり出回っていません。そのため、C#は主に、すでにこの言語を使い慣れている経験豊富なユーザーにお勧めしています。
各言語の特徴や長所・短所を見ていきましょう。
GDScript¶
GDScript は、オブジェクト指向および `命令型 <https://ja.wikipedia.org/wiki/%E5%91%BD%E4%BB%A4%E5%9E%8B%E3%83%97%E3%83%AD%E3%82%B0%E3%83%A9%E3%83%9F%E3%83%B3%E3%82%B0`_プログラミング言語であり、Godot用に開発されました。ゲーム開発者のために、ゲームコーディングの時間を短縮するために作られました。その特徴は以下の通りです。
短いファイルにつながるシンプルな構文。
コンパイルと読み込みが非常に速い。
エディタとの緊密な統合により、ノードやシグナルなどの情報をシーンからコード補完することができます。
ベクトル型と変換型を内蔵しており、ゲームに必須の線形代数の多用に効率的です。
静的型付き言語と同じようにマルチスレッドに対応しています。
ガベージ コレクション はありません。この機能はゲーム作成時に結局邪魔になるので、この機能は使用できません。デフォルトでは、ほとんどの場合、エンジンが参照をカウントしてメモリを管理してくれますが、必要であればメモリを制御することもできます。
Gradual typingです。変数はデフォルトで動的な型を持ちますが、型ヒントを用いて強力な型チェックを行うこともできます。
GDScript はインデントを使ってコードブロックを構成するため Python のように見えますが、実際には同じようには動きません。GDScriptは、Squirrel、Lua、Pythonなど複数の言語からヒントを得ています。
注釈
なぜ我々はPythonやLuaを直接使っていないのか?
数年前、GodotはPythonを使い、次にLuaを使いました。どちらの言語も、統合には多くの労力を要し、大きな制約がありました。例えば、Pythonではスレッドのサポートが大きな課題でした。
専用言語を開発することで手間がかからず、ゲーム開発者のニーズに合わせてカスタマイズすることができます。現在、サードパーティーの言語では提供することが難しかったパフォーマンスの最適化や機能の開発に取り組んでいます。
.NET / C#¶
As Microsoft's C# is a favorite amongst game developers, we officially support it. C# is a mature and flexible language with tons of libraries written for it. We were able to add support for it thanks to a generous donation from Microsoft.
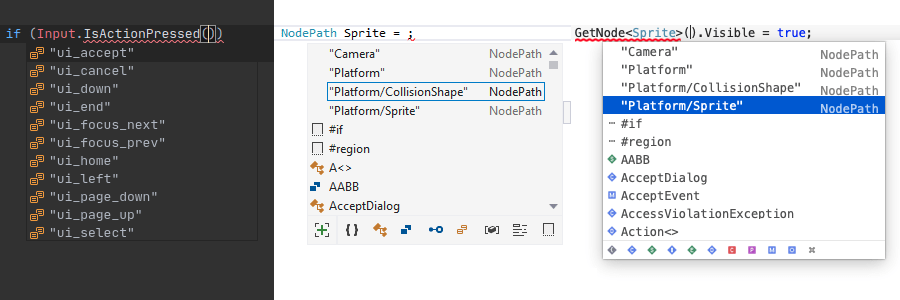
C#は、ガベージコレクタに注意する必要がありますが、性能と使いやすさの間のトレードオフを提供します。
注釈
You must use the .NET edition of the Godot editor to script in C#. You can download it on the Godot website's download page.
Since Godot uses .NET 6, in theory, you can use any third-party .NET library or framework in Godot, as well as any Common Language Infrastructure-compliant programming language, such as F#, Boo, or ClojureCLR. However, C# is the only officially supported .NET option.
注釈
GDScriptコード自体は、コンパイルされたC#またはC++ほど高速には実行されません。ただし、ほとんどのスクリプトコードは、エンジン内のC++コードで記述された高速なアルゴリズムの関数を呼び出します。多くの場合、GDScript、C#、またはC ++でゲームプレイロジックを記述しても、パフォーマンスに大きな影響はありません。
注意
Projects written in C# using Godot 4 currently cannot be exported to the web platform. To use C# on that platform, consider Godot 3 instead. Android and iOS platform support is available as of Godot 4.2, but is experimental and some limitations apply.
C++ via GDExtension¶
GDExtension allows you to write game code in C++ without needing to recompile Godot.
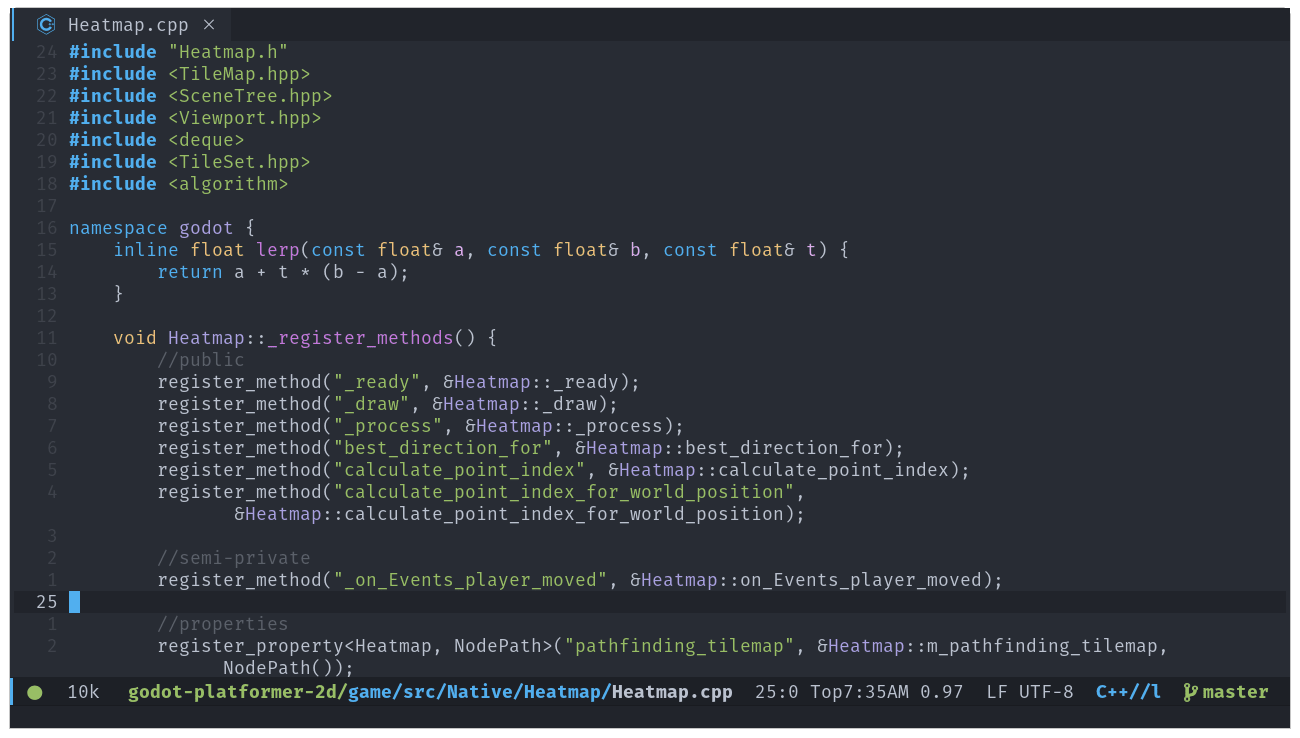
C API Bridgeの利用により、生成される共有ライブラリには、任意の言語のバージョンやコンパイラブランド、バージョンの混在が可能です。
GDExtension is the best choice for performance. You don't need to use it throughout an entire game, as you can write other parts in GDScript or C#.
When working with GDExtension, the available types, functions, and properties closely resemble Godot's actual C++ API.
概要¶
スクリプトとは、機能を拡張したいノードに接続されるコードを含むファイルです。
Godot supports four official scripting languages, offering you flexibility between performance and ease of use.
必要なアルゴリズムをCやC++で実装してほとんどのゲームロジックをGDScriptやC#で書く、というような言語を混ぜて開発することも可能です。