Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
RichTextLabelのBBCode¶
はじめに¶
Label nodes are great for displaying basic text, but they have limitations. If you want to change the color of the text, or its alignment, you can only do that to the entire label. You can't make a part of the text have another color, or have a part of the text centered. To get around these limitations, you would use a RichTextLabel.
RichTextLabel allows for complex formatting of text using a markup syntax or the built-in API. It uses BBCodes for the markup syntax, a system of tags that designate formatting rules for a part of the text. You may be familiar with them if you ever used forums (also known as bulletin boards, hence the "BB" in "BBCode").
Unlike Label, RichTextLabel also comes with its own vertical scrollbar. This scrollbar is automatically displayed if the text does not fit within the control's size. The scrollbar can be disabled by unchecking the Scroll Active property in the RichTextLabel inspector.
Note that the BBCode tags can also be used to some extent in the XML source of the class reference. For more information, see Class reference primer.
参考
You can see how BBCode in RichTextLabel works in action using the Rich Text Label with BBCode demo project.
BBCodeを使用する¶
By default, RichTextLabel functions like a normal Label. It has the property_text property, which you can edit to have uniformly formatted text. To be able to use BBCode for rich text formatting, you need to turn on the BBCode mode by setting bbcode_enabled. After that, you can edit the text property using available tags. Both properties are located at the top of the inspector after selecting a RichTextLabel node.
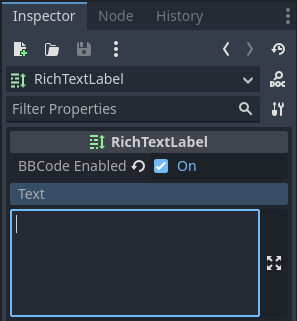
For example, BBCode [color=green]test[/color]
would render the word "test" with
a green color.
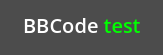
Most BBCodes consist of 3 parts: the opening tag, the content and the closing
tag. The opening tag delimits the start of the formatted part, and can also
carry some configuration options. Some opening tags, like the color
one
shown above, also require a value to work. Other opening tags may accept
multiple options (separated by spaces within the opening tag). The closing tag
delimits the end of the formatted part. In some cases, both the closing tag and
the content can be omitted.
Unlike BBCode in HTML, leading/trailing whitespace is not removed by a RichTextLabel upon display. Duplicate spaces are also displayed as-is in the final output. This means that when displaying a code block in a RichTextLabel, you don't need to use a preformatted text tag.
[tag]content[/tag]
[tag=value]content[/tag]
[tag option1=value1 option2=value2]content[/tag]
[tag][/tag]
[tag]
注釈
RichTextLabel doesn't support entangled BBCode tags. For example, instead of using:
[b]bold[i]bold italic[/b]italic[/i]
Use:
[b]bold[i]bold italic[/i][/b][i]italic[/i]
Handling user input safely¶
In a scenario where users may freely input text (such as chat in a multiplayer
game), you should make sure users cannot use arbitrary BBCode tags that will be
parsed by RichTextLabel. This is to avoid inappropriate use of formatting, which
can be problematic if [url]
tags are handled by your RichTextLabel (as players
may be able to create clickable links to phishing sites or similar).
Using RichTextLabel's [lb]
and/or [rb]
tags, we can replace the opening and/or
closing brackets of any BBCode tag in a message with those escaped tags. This
prevents users from using BBCode that will be parsed as tags – instead, the
BBCode will be displayed as text.

Example of unescaped user input resulting in BBCode injection (2nd line) and escaped user input (3rd line)¶
The above image was created using the following script:
extends RichTextLabel
func _ready():
append_chat_line("Player 1", "Hello world!")
append_chat_line("Player 2", "Hello [color=red]BBCode injection[/color] (no escaping)!")
append_chat_line_escaped("Player 2", "Hello [color=red]BBCode injection[/color] (with escaping)!")
# Returns escaped BBCode that won't be parsed by RichTextLabel as tags.
func escape_bbcode(bbcode_text):
# We only need to replace opening brackets to prevent tags from being parsed.
return bbcode_text.replace("[", "[lb]")
# Appends the user's message as-is, without escaping. This is dangerous!
func append_chat_line(username, message):
append_text("%s: [color=green]%s[/color]\n" % [username, message])
# Appends the user's message with escaping.
# Remember to escape both the player name and message contents.
func append_chat_line_escaped(username, message):
append_text("%s: [color=green]%s[/color]\n" % [escape_bbcode(username), escape_bbcode(message)])
パフォーマンス¶
In most cases, you can use BBCode directly as-is since text formatting is rarely a heavy task. However, with particularly large RichTextLabels (such as console logs spanning thousands of lines), you may encounter stuttering during gameplay when the RichTextLabel's text is updated.
There are several ways to alleviate this:
Use the
append_text()
function instead of appending to thetext
property. This function will only parse BBCode for the added text, rather than parsing BBCode from the entiretext
property.Use
push_[tag]()
andpop()
functions to add tags to RichTextLabel instead of using BBCode.Enable the Threading > Threaded property in RichTextLabel. This won't speed up processing, but it will prevent the main thread from blocking, which avoids stuttering during gameplay. Only enable threading if it's actually needed in your project, as threading has some overhead.
Using push_[tag]() and pop() functions instead of BBCode¶
If you don't want to use BBCode for performance reasons, you can use functions provided by RichTextLabel to create formatting tags without writing BBCode in the text.
Every BBCode tag (including effects) has a push_[tag]()
function (where
[tag]
is the tag's name). There are also a few convenience functions
available, such as push_bold_italics()
that combines both push_bold()
and push_italics()
into a single tag. See the
RichTextLabel class reference for a complete list of
push_[tag]()
functions.
The pop()
function is used to end any tag. Since BBCode is a tag stack,
using pop()
will close the most recently started tags first.
The following script will result in the same visual output as using
BBCode [color=green]test [i]example[/i][/color]
:
extends RichTextLabel
func _ready():
append_text("BBCode ") # Trailing space separates words from each other.
push_color(Color.GREEN)
append_text("test ") # Trailing space separates words from each other.
push_italics()
append_text("example")
pop() # Ends the tag opened by `push_italics()`.
pop() # Ends the tag opened by `push_color()`.
警告
Do not set the text
property directly when using formatting functions.
Appending to the text
property will erase all modifications made to the
RichTextLabel using the append_text()
, push_[tag]()
and pop()
functions.
リファレンス¶
タグ |
例 |
b
Makes
{text} use the bold (or bold italics) font of RichTextLabel . |
|
i
Makes
{text} use the italics (or bold italics) font of RichTextLabel . |
|
u
Makes
{text} underlined. |
|
s
Makes
{text} strikethrough. |
|
コード
Makes
{text} use the mono font of RichTextLabel . |
|
p
Adds new paragraph with
{text} . Supports configuration options,
see Paragraph options. |
[p]{text}[/p] [p {options}]{text}[/p] |
センター
Makes
{text} horizontally centered.Same as
[p align=center] . |
|
left
Makes
{text} horizontally left-aligned.Same as
[p align=left] . |
|
右
Makes
{text} horizontally right-aligned.Same as
[p align=right] . |
|
フィル
Makes
{text} fill the full width of RichTextLabel .Same as
[p align=fill] . |
|
インデント
Indents
{text} once.
The indentation width is the same as with [ul] or [ol] , but without a bullet point. |
|
** URL **
Creates a hyperlink (underlined and clickable text). Can contain optional
{text} or display {link} as is.Must be handled with the "meta_clicked" signal to have an effect, see Handling [url] tag clicks.
|
[url]{link}[/url] [url={link}]{text}[/url] |
hint
Creates a tooltip hint that is displayed when hovering the text with the mouse.
Tooltip text should not be quoted (quotes will appear as-is in the tooltip otherwise).
|
[hint={tooltip text displayed on hover}]{text}[/hint] |
img
Inserts an image from the
{path} (can be any valid Texture2D resource).If
{width} is provided, the image will try to fit that width maintaining
the aspect ratio.If both
{width} and {height} are provided, the image will be scaled
to that size.If
{valign} configuration is provided, the image will try to align to the
surrounding text, see Image vertical alignment.Supports configuration options, see Image options.
|
[img]{path}[/img] [img={width}]{path}[/img] [img={width}x{height}]{path}[/img] [img={valign}]{path}[/img] [img {options}]{path}[/img] |
フォント
Makes
{text} use a font resource from the {path} .Supports configuration options, see Font options.
|
[font={path}]{text}[/font] [font {options}]{text}[/font] |
font_size
Use custom font size for
{text} . |
|
dropcap
Use a different font size and color for
{text} , while making the tag's contents
span multiple lines if it's large enough.A drop cap is typically one
uppercase character, but
[dropcap] supports containing multiple characters.
margins values are comma-separated and can be positive, zero or negative.
Negative top and bottom margins are particularly useful to allow the rest of
the paragraph to display below the dropcap. |
|
opentype_features
Enables custom OpenType font features for
{text} . Features must be provided as
a comma-separated {list} . |
[opentype_features={list}] {text} [/opentype_features] |
lang
Overrides the language for
{text} that is set by the BiDi > Language property
in RichTextLabel. {code} must be an ISO language code.
This can be used to enforce the use of a specific script for a language without
starting a new paragraph. Some font files may contain script-specific substitutes,
in which case they will be used. |
|
色
Changes the color of
{text} . Color must be provided by a common name (see
Named colors) or using the HEX format (e.g.
#ff00ff , see 16進カラーコード). |
|
bgcolor
Draws the color behind
{text} . This can be used to highlight text.
Accepts same values as the color tag. |
|
fgcolor
Draws the color in front of
{text} . This can be used to "redact" text by using
an opaque foreground color. Accepts same values as the color tag. |
|
outline_size
Use custom font outline size for
{text} . |
[outline_size={size}] {text} [/outline_size] |
outline_color
Use custom outline color for
{text} . Accepts same values as the color tag. |
[outline_color={code/name}] {text} [/outline_color] |
table
Creates a table with the
{number} of columns. Use the cell tag to define
table cells. |
|
cell
Adds a cell with
{text} to the table.If
{ratio} is provided, the cell will try to expand to that value proportionally
to other cells and their ratio values.Supports configuration options, see Cell options.
|
[cell]{text}[/cell] [cell={ratio}]{text}[/cell] [cell {options}]{text}[/cell] |
ul
Adds an unordered list. List
{items} must be provided by putting one item per
line of text.The bullet point can be customized using the
{bullet} parameter,
see Unordered list bullet. |
[ul]{items}[/ul] [ul bullet={bullet}]{items}[/ul] |
ol
Adds an ordered (numbered) list of the given
{type} (see Ordered list types).
List {items} must be provided by putting one item per line of text. |
|
lb, rb
Adds
[ and ] respectively. Allows escaping BBCode markup.These are self-closing tags, which means you do not need to close them
(and there is no
[/lb] or [/rb] closing tag). |
[lb]b[rb]text[lb]/b[rb] will display as [b]text[/b] . |
Several Unicode control characters can be added using their own self-closing tags.
This can result in easier maintenance compared to pasting those
control characters directly in the text.
|
[lrm] (left-to-right mark), [rlm] (right-to-left mark), [lre] (left-to-right embedding),[rle] (right-to-left embedding), [lro] (left-to-right override), [rlo] (right-to-left override),[pdf] (pop directional formatting), [alm] (Arabic letter mark), [lri] (left-to-right isolate),[rli] (right-to-left isolate), [fsi] (first strong isolate), [pdi] (pop directional isolate),[zwj] (zero-width joiner), [zwnj] (zero-width non-joiner), [wj] (word joiner),[shy] (soft hyphen) |
注釈
Tags for bold ([b]
) and italics ([i]
) formatting work best if the
appropriate custom fonts are set up in the RichTextLabelNode's theme
overrides. If no custom bold or italic fonts are defined,
faux bold and italic fonts
will be generated by Godot. These fonts rarely look good in comparison to hand-made bold/italic font variants.
The monospaced ([code]
) tag only works if a custom font is set up in
the RichTextLabel node's theme overrides. Otherwise, monospaced text will use the regular font.
There are no BBCode tags to control vertical centering of text yet.
Options can be skipped for all tags.
Paragraph options¶
align
Values
left
,center
,right
,fill
Default
left
Text horizontal alignment.
bidi_override, st
Values
default
,uri
,file
,email
,list
,none
,custom
Default
default
Structured text override.
direction, dir
Values
ltr
,rtl
,auto
Default
継承
Base BiDi direction.
language, lang
Values
ISO language codes. See Locale codes
Default
継承
Locale override. Some font files may contain script-specific substitutes, in which case they will be used.
tab_stops
Values
List of floating-point numbers, e.g.
10.0,30.0
Default
Width of the space character in the font
Overrides the horizontal offsets for each tab character. When the end of the list is reached, the tab stops will loop over. For example, if you set
tab_stops
to10.0,30.0
, the first tab will be at10
pixels, the second tab will be at10 + 30 = 40
pixels, and the third tab will be at10 + 30 + 10 = 50
pixels from the origin of the RichTextLabel.
Handling [url]
tag clicks¶
By default, [url]
tags do nothing when clicked. This is to allow flexible use
of [url]
tags rather than limiting them to opening URLs in a web browser.
To handle clicked [url]
tags, connect the RichTextLabel
node's
meta_clicked signal to a script function.
For example, the following method can be connected to meta_clicked
to open
clicked URLs using the user's default web browser:
# This assumes RichTextLabel's `meta_clicked` signal was connected to
# the function below using the signal connection dialog.
func _richtextlabel_on_meta_clicked(meta):
# `meta` is not guaranteed to be a String, so convert it to a String
# to avoid script errors at run-time.
OS.shell_open(str(meta))
For more advanced use cases, it's also possible to store JSON in an [url]
tag's option and parse it in the function that handles the meta_clicked
signal.
For example:
[url={"example": "value"}]JSON[/url]
Image options¶
色
Values
Color name or color in HEX format
Default
継承
Color tint of the image (modulation).
height
Values
Number in pixels
Default
継承
Target height of the image.
width
Values
Number in pixels
Default
継承
Target width of the image.
region
Values
x,y,width,height in pixels
Default
継承
Region rect of the image. This can be used to display a single image from a spritesheet.
Image vertical alignment¶
When a vertical alignment value is provided with the [img]
tag the image
will try to align itself against the surrounding text. Alignment is performed
using a vertical point of the image and a vertical point of the text. There are
3 possible points on the image (top
, center
, and bottom
) and 4
possible points on the text (top
, center
, baseline
, and bottom
),
which can be used in any combination.
To specify both points, use their full or short names as a value of the image tag:
[img=top,bottom]
[img=center,center]
You can also specify just one value (top
, center
, or bottom
) to make
use of a corresponding preset (top-top
, center-center
, and bottom-bottom
respectively).
Short names for the values are t
(top
), c
(center
), l
(baseline
),
and b
(bottom
).
Font options¶
name, n
Values
A valid Font resource path.
Default
継承
Font resource path.
size, s
Values
Number in pixels.
Default
継承
Custom font size.
Named colors¶
For tags that allow specifying a color by name you can use names of the constants from
the built-in Color class. Named classes can be specified in a number of
styles using different casings: DARK_RED
, DarkRed
, and darkred
will give
the same exact result.
16進カラーコード¶
For opaque RGB colors, any valid 6-digit hexadecimal code is supported, e.g.
[color=#ffffff]white[/color]
. Shorthand RGB color codes such as #6f2
(equivalent to #66ff22
) are also supported.
For transparent RGB colors, any RGBA 8-digit hexadecimal code can be used,
e.g. [color=#ffffff88]translucent white[/color]
. Note that the alpha channel
is the last component of the color code, not the first one. Short RGBA
color codes such as #6f28
(equivalent to #66ff2288
) are supported as well.
Cell options¶
expand
Values
Integer number
Default
1
Cell expansion ratio. This defines which cells will try to expand to proportionally to other cells and their expansion ratios.
border
Values
Color name or color in HEX format
Default
継承
Cell border color.
bg
Values
Color name or color in HEX format
Default
継承
Cell background color. For alternating odd/even row backgrounds, you can use
bg=odd_color,even_color
.
Unordered list bullet¶
By default, the [ul]
tag uses the U+2022
"Bullet" Unicode glyph as the
bullet character. This behavior is similar to web browsers. The bullet character
can be customized using [ul bullet={bullet}]
. If provided, this {bullet}
parameter must be a single character with no enclosing quotes (for example,
[bullet=*]
). Additional characters are ignored. The bullet character's
width does not affect the list's formatting.
See Bullet (typography) on Wikipedia
for a list of common bullet characters that you can paste directly in the bullet
parameter.
Ordered list types¶
Ordered lists can be used to automatically mark items with numbers or letters in ascending order. This tag supports the following type options:
1
- Numbers, using language specific numbering system if possible.a
,A
- Lower and upper case Latin letters.i
,I
- Lower and upper case Roman numerals.
Text effects¶
BBCode can also be used to create different text effects that can optionally be animated. Five customizable effects are provided out of the box, and you can easily create your own. By default, animated effects will pause when the SceneTree is paused. You can change this behavior by adjusting the RichTextLabel's Process > Mode property.
All examples below mention the default values for options in the listed tag format.
注釈
Text effects that move characters' position may result in characters being clipped by the RichTextLabel node bounds.
You can resolve this by disabling Control > Layout > Clip Contents in the inspector after selecting the RichTextLabel node, or ensuring there is enough margin added around the text by using line breaks above and below the line using the effect.
Pulse¶
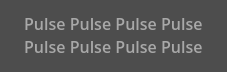
Pulse creates an animated pulsing effect that multiplies each character's
opacity and color. It can be used to bring attention to specific text. Its tag
format is [pulse freq=1.0 color=#ffffff40 ease=-2.0]{text}[/pulse]
.
freq
controls the frequency of the half-pulsing cycle (higher is faster). A
full pulsing cycle takes 2 * (1.0 / freq)
seconds. color
is the target
color multiplier for blinking. The default mostly fades out text, but not
entirely. ease
is the easing function exponent to use. Negative values
provide in-out easing, which is why the default is -2.0
.
Wave(波)¶
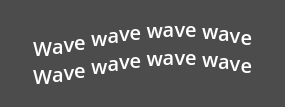
Wave makes the text go up and down. Its tag format is
[wave amp=50.0 freq=5.0 connected=1]{text}[/wave]
.
amp
controls how high and low the effect goes, and freq
controls how
fast the text goes up and down. A freq
value of 0
will result in no
visible waves, and negative freq
values won't display any waves either. If
connected
is 1
(default), glyphs with ligatures will be moved together.
If connected
is 0
, each glyph is moved individually even if they are
joined by ligatures. This can work around certain rendering issues with font
ligatures.
Tornado(竜巻)¶
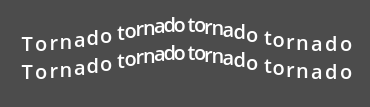
Tornado makes the text move around in a circle. Its tag format is
[tornado radius=10.0 freq=1.0 connected=1]{text}[/tornado]
.
radius
is the radius of the circle that controls the offset, freq
is how
fast the text moves in a circle. A freq
value of 0
will pause the
animation, while negative freq
will play the animation backwards. If
connected
is 1
(default), glyphs with ligatures will be moved together.
If connected
is 0
, each glyph is moved individually even if they are
joined by ligatures. This can work around certain rendering issues with font
ligatures.
Shake(シェイク)¶
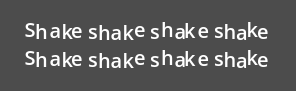
Shake makes the text shake. Its tag format is
[shake rate=20.0 level=5 connected=1]{text}[/shake]
.
rate
controls how fast the text shakes, level
controls how far the text
is offset from the origin. If connected
is 1
(default), glyphs with
ligatures will be moved together. If connected
is 0
, each glyph is moved
individually even if they are joined by ligatures. This can work around certain
rendering issues with font ligatures.
Fade(フェード)¶
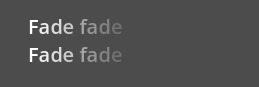
Fade creates a static fade effect that multiplies each character's opacity.
Its tag format is [fade start=4 length=14]{text}[/fade]
.
start
controls the starting position of the falloff relative to where the fade
command is inserted, length
controls over how many characters should the fade
out take place.
Rainbow(虹)¶
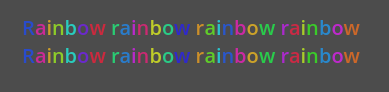
Rainbow gives the text a rainbow color that changes over time. Its tag format is
[rainbow freq=1.0 sat=0.8 val=0.8]{text}[/rainbow]
.
freq
is the number of full rainbow cycles per second, sat
is the
saturation of the rainbow, val
is the value of the rainbow. A freq
value
of 0
will pause the animation, while negative freq
will play the
animation backwards.
Font outlines are not affected by the rainbow effect (they keep their original color). Existing font colors are overridden by the rainbow effect. However, CanvasItem's Modulate and Self Modulate properties will affect how the rainbow effect looks, as modulation multiplies its final colors.