Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
暂停游戏与处理模式¶
前言¶
在大多数游戏中,或多或少都会希望能够中断游戏去干些别的,例如休息一下或者修改选项。要非常细致地控制哪些可以暂停(哪些不可以)实现起来非常麻烦,所以 Godot 提供了一个简单的暂停框架。
如何暂停工作¶
要将游戏暂停,就必须设置暂停状态。将 SceneTree.paused 属性赋值为 true
即可:
get_tree().paused = true
GetTree().Paused = true;
这样做会导致两件事。首先,所有节点的 2D 和 3D 物理都会停止。其次,根据处理模式的不同,某些节点的行为会停止或者开始。
备注
游戏暂停时,可以通过 set_active
方法激活物理服务器。
处理模式¶
Each node in Godot has a "Process Mode" that defines when it processes. It can be found and changed under a node's Node properties in the inspector.
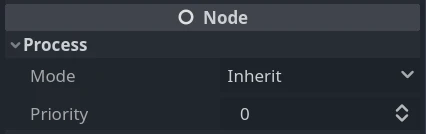
你也可以通过代码来修改该属性:
func _ready():
process_mode = Node.PROCESS_MODE_PAUSABLE
public override void _Ready()
{
ProcessMode = Node.ProcessModeEnum.Pausable;
}
各个模式对节点的要求是这样的:
Inherit(继承) :处理与否取决于父、祖父等等节点中第一个具有非 Inherit 状态的节点。
Pausable: Process the node (and its children in Inherit mode) only when the game is not paused.
WhenPaused: Process the node (and its children in Inherit mode) only when the game is paused.
Always: Process the node (and its children in Inherit mode) no matter what. Paused or not, this node will process.
Disabled: The node (and its children in Inherit mode) will not process at all.
默认情况下,所有节点的这个属性都是“Inherit”状态。如果父节点也是“Inherit”,那么就会去检查祖父节点,以此类推。如果祖宗们都没有更改过状态,那么就会使用 SceneTree 的暂停状态。也就是说,在游戏暂停时默认所有节点都会被暂停下来。节点停止处理时会发生不少事情。
The _process
, _physics_process
, _input
, and _input_event
functions
will not be called. However signals still work and cause their connected function to
run, even if that function's script is attached to a node that is not currently being processed.
动画节点会暂停它们的当前动画,音频节点会暂停它们的当前音频流,粒子也会暂停。游戏不再暂停时,它们都会自动继续运行。
请注意有一点非常重要,即便游戏暂停时节点仍在进行处理,但物理默认是无法正常工作的。如前文所述,这是因为物理服务器会被关闭。游戏暂停时,可以通过 set_active
方法激活物理服务器。