Up to date
This page is up to date for Godot 4.2
.
If you still find outdated information, please open an issue.
Перетворення вікна перегляду та полотна¶
Вступ¶
Це огляд 2D-перетворень, що відбуваються з вузлами від моменту, коли вони малюють свій вміст локально, до того часу, коли вони потрапляють на екран. У цьому огляді розглядаються деталі движка на дуже низькому рівні.
The goal of this tutorial is to teach a way for feeding input events to the Input with a position in the correct coordinate system.
A more extensive description of all coordinate systems and 2d transforms is available in 2D coordinate systems and 2D transforms.
Перетворення полотна¶
Як уже згадувалося в попередньому посібнику Полотняні шари, кожен вузол CanvasItem (пам’ятайте, що вузли на основі Node2D та Control використовують CanvasItem як загальний корінь) буде знаходитись у Шарі Полотна. Кожен шар полотна має перетворення (переміщення, обертання, масштаб тощо), до якого можна отримати доступ як Transform2D.
Також в попередньому посібнику говорилося, що вузли за замовчуванням малюються на рівні 0 на вбудованому полотні. Щоб розмістити вузли в іншому шарі, можна використовувати вузол CanvasLayer (Полотняні Шари).
Глобальне перетворення полотна¶
Viewports also have a Global Canvas transform (also a Transform2D). This is the master transform and affects all individual Canvas Layer transforms. Generally, this is primarily used in Godot's CanvasItem Editor.
Перетворення розтягування¶
Нарешті, у вікна перегляду є Перетворення Розтягування, яке використовується при зміні розміру або розтягуванні екрана. Це перетворення використовується внутрішньо (як описано в розділі Multiple resolutions), але його також можна встановити вручну для кожного вікна перегляду.
Input events are multiplied by this transform but lack the ones above. To convert InputEvent coordinates to local CanvasItem coordinates, the CanvasItem.make_input_local() function was added for convenience.
Window transform¶
The root viewport is a Window. In order to scale and position the Window's content as described in Multiple resolutions, each Window contains a window transform. It is for example responsible for the black bars at the Window's sides so that the Viewport is displayed with a fixed aspect ratio.
Порядок перетворень¶
To convert a CanvasItem local coordinate to an actual screen coordinate, the following chain of transforms must be applied:
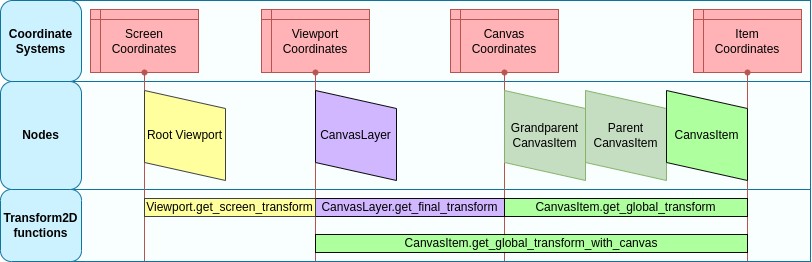
Функції перетворення¶
The above graphic shows some available transform functions. All transforms are directed from right to left, this means multiplying a transform with a coordinate results in a coordinate system further to the left, multiplying the affine inverse of a transform results in a coordinate system further to the right:
# Called from a CanvasItem.
canvas_pos = get_global_transform() * local_pos
local_pos = get_global_transform().affine_inverse() * canvas_pos
// Called from a CanvasItem.
canvasPos = GetGlobalTransform() * localPos;
localPos = GetGlobalTransform().AffineInverse() * canvasPos;
Нарешті, щоб перетворити локальні координати CanvasItem на екранні координати, просто помножте їх у наступному порядку:
var screen_coord = get_viewport().get_screen_transform() * get_global_transform_with_canvas() * local_pos
var screenCord = GetViewport().GetScreenTransform() * GetGlobalTransformWithCanvas() * localPos;
Однак майте на увазі, що, як правило, не бажано працювати з координатами екрану. Рекомендований підхід полягає в тому, щоб просто працювати в координатах Полотна (CanvasItem.get_global_transform()
), щоб дозволити автоматичній зміні розміру роздільної здатності екрана працювати належним чином.
Подача власних подій введення¶
It is often desired to feed custom input events to the game. With the above knowledge, to correctly do this in the focused window, it must be done the following way:
var local_pos = Vector2(10, 20) # Local to Control/Node2D.
var ie = InputEventMouseButton.new()
ie.button_index = MOUSE_BUTTON_LEFT
ie.position = get_viewport().get_screen_transform() * get_global_transform_with_canvas() * local_pos
Input.parse_input_event(ie)
var localPos = new Vector2(10,20); // Local to Control/Node2D.
var ie = new InputEventMouseButton()
{
ButtonIndex = MouseButton.Left,
Position = GetViewport().GetScreenTransform() * GetGlobalTransformWithCanvas() * localPos,
};
Input.ParseInputEvent(ie);